- What is the Bitwise AND Operator?
- What Does “X&3” Mean?
- Evaluating “X&3” in Various Scenarios
- Why Does “X&3” Work So Effectively?
- Common Use Cases for “X&3”
- Conclusion
- FAQs
- What does the Bitwise AND operation with 3 do?
- Why use the Bitwise AND operation with 3?
- What is the result of applying the Bitwise AND operation between 6 and 3?
- Can the Bitwise AND operation with 3 check if a number is even?
- What type of numbers can the Bitwise AND operation with 3 be applied to?
- Where is the Bitwise AND operation with 3 commonly used?
In the world of programming, bitwise operators are fundamental tools that manipulate individual bits within a number.
Among these operators, the Bitwise AND operator is widely used. One specific expression you may encounter is “X&3”, which involves applying the AND operator to a variable and the number 3.
In this article, we will break down the concept of the Bitwise AND operation and understand how “X&3” works in various contexts.
What is the Bitwise AND Operator?
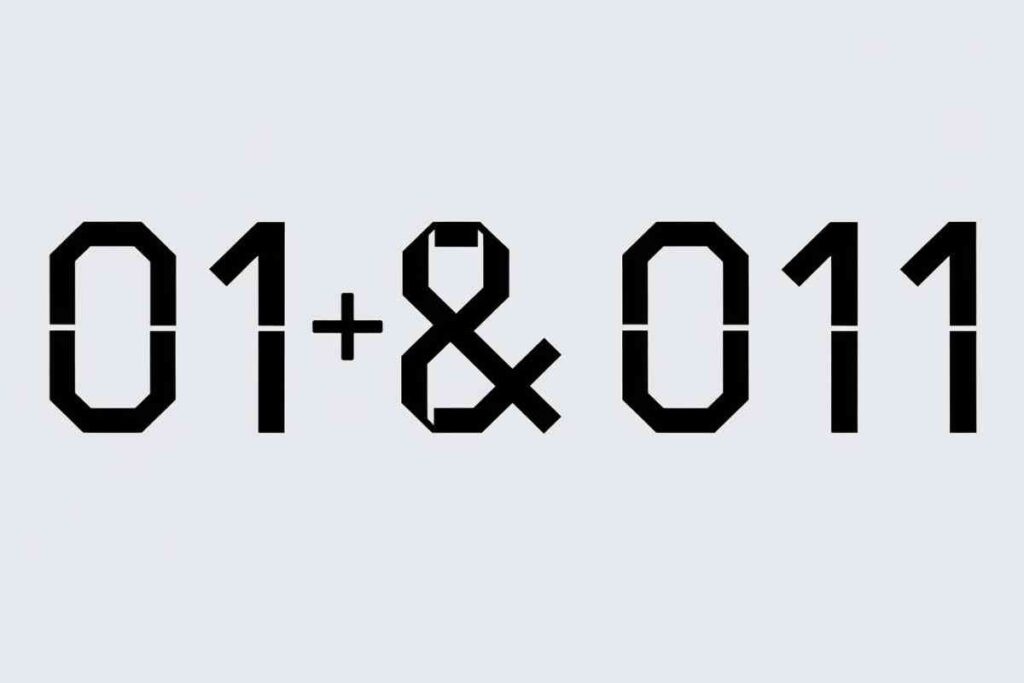
The Bitwise AND operator, represented by the symbol & , is used to compare each corresponding bit of two numbers.
For each bit pair, if both bits are 1, the result will be 1; otherwise, the result will be 0. This operation is applied bit by bit on the binary representation of the numbers.
How the Bitwise AND Operator Works
- If both bits are
1
, the result is1
. - If one bit is
0
and the other is1
, the result is0
. - If both bits are
0
, the result is0
.
This might sound complex, but it becomes clear once you look at an example:
For example, let’s consider the numbers 6
and 3
:
6
in binary is0110
3
in binary is0011
When we apply 6 & 3
, the comparison is done bit by bit:
sqlCopy code 0110 (6 in binary)
& 0011 (3 in binary)
---------
0010 (2 in binary)
So, 6 & 3
results in 2
.
What Does “X&3” Mean?
The expression “X&3” simply refers to applying the Bitwise AND operator between the value of x and the number 3.
Understanding the Operand “3” in “X&3”
The number 3 in binary is 0011. This means that when you perform the operation x AND 3, you are effectively masking all but the last two bits of the variable v.
This is because 3 (in binary 0011) has its two rightmost bits set to 1, which means only those bits from x are preserved in the result.
What Happens to X?
- If
X
is an integer, applyingX&3
will extract the last two bits ofX
. - For example, if
X = 5
:5
in binary is0101
- Applying
5 & 3
:sqlCopy code0101 (5 in binary) & 0011 (3 in binary) -------- 0001 (1 in binary)
The result is1
, which is the value of the last two bits ofX
(5).
Why Use “X&3”?
The operation “X&3” is commonly used when you want to extract specific bits from a number. This operation helps in scenarios such as:
- Extracting even or odd numbers.
- Masking values to select specific bits for processing.
- Efficient operations in performance-critical applications, such as embedded systems.
Evaluating “X&3” in Various Scenarios
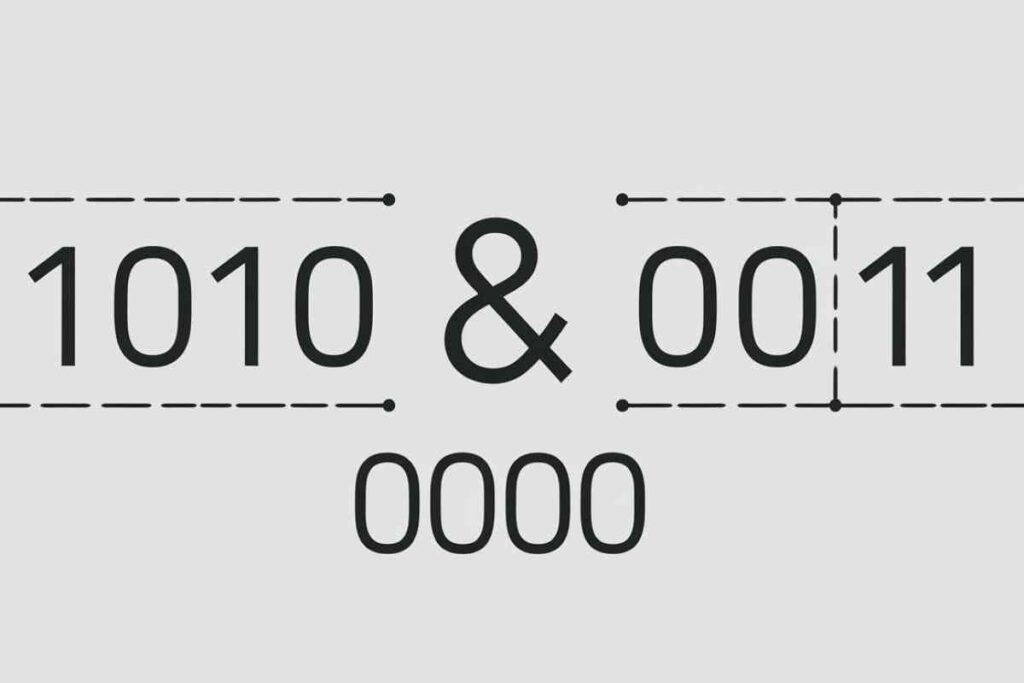
Let’s explore more examples of “X&3” in different scenarios. This will help you understand how it works with different numbers and types of data.
Example 1: X = 6
- Binary of
6
:0110
- Applying
X&3
:markdownCopy code0110 & 0011 -------- 0010 (Result: 2)
Example 2: X = 15
- Binary of
15
:1111
- Applying
X&3
:markdownCopy code1111 & 0011 -------- 0011 (Result: 3)
Example 3: X = 22
- Binary of
22
:10110
- Applying
X&3
:markdownCopy code10110 & 0011 -------- 0010 (Result: 2)
In these examples, the operation extracts the last two bits of X
, allowing us to work with a smaller set of values, which is especially useful in situations where we only care about certain bits of a number.
Why Does “X&3” Work So Effectively?
The reason “X&3” is widely used is because of the properties of binary numbers and the efficiency of bitwise operations.
Bitwise operations are very fast and low-level operations that work directly on the bits of a number, making them ideal for tasks that require high performance and quick computation.
- Efficiency: Bitwise operations are much faster than traditional arithmetic operations.
- Memory Saving: By focusing on specific bits, we can often reduce memory usage.
- Bitmasking: It is commonly used in systems programming, especially in low-level programming where access to specific bits is crucial.
Common Use Cases for “X&3”

- Extracting Values from Bit Fields In many systems, data is stored in bit fields where each bit or group of bits has a specific meaning. The expression “X&3” allows developers to quickly extract the lower bits to check or manipulate specific values.
- Checking Even or Odd Numbers By performing a Bitwise AND with
1
, you can check if a number is even or odd. Similarly, using3
allows extraction of a set of bits for custom checks. - Data Masking Masking operations involve preserving certain bits and setting others to zero. This is useful in applications such as encryption, image processing, and low-level hardware programming.
Conclusion
In conclusion, “X&3” is a bitwise operation that extracts the last two bits of a number. It’s a highly efficient technique used in various programming tasks, such as bitmasking and checking specific bit patterns.
Understanding this operation is essential for low-level programming and performance optimization.
FAQs
What does the Bitwise AND operation with 3 do?
It applies the Bitwise AND operator to a number and 3, isolating the last two bits of the number.
Why use the Bitwise AND operation with 3?
It helps extract the last two bits of a number quickly and efficiently.
What is the result of applying the Bitwise AND operation between 6 and 3?
The result is 2 because the binary representation of 6 and 3 produces 0010.
Can the Bitwise AND operation with 3 check if a number is even?
No, the operation checks the last two bits, which doesn’t directly tell if a number is even.
What type of numbers can the Bitwise AND operation with 3 be applied to?
It can be applied to integers, as the Bitwise AND operation works on integral types.
Where is the Bitwise AND operation with 3 commonly used?
It’s commonly used in systems programming, performance optimization, and bit manipulation tasks.