- What is Timber get posts tax_query WordPress?
- How Timber Simplifies WordPress Queries?
- Using Timber::get_posts with tax_query
- Syntax Breakdown of Timber get posts tax_query
- Filtering by Multiple Taxonomies
- Practical Examples of Timber get posts tax_query
- Common Issues and Troubleshooting Tips
- Best Practices for SEO with Timber get posts tax_query
- Conclusion
- FAQs
- What is Timber get posts tax_query in WordPress?
- How does Timber simplify tax_query usage?
- Can I filter by custom taxonomies in Timber?
- What are the main tax_query parameters?
- How do I filter posts by multiple taxonomies?
- What happens if I use an incorrect taxonomy in tax_query?
- Does tax_query affect performance on large sites?
- Can I combine tax_query with other query parameters in Timber?
WordPress has always been a robust platform for building websites, but with the rise of modern development practices, frameworks like Timber have made WordPress development more flexible, efficient, and user-friendly.
If you’re working with custom themes or need to fetch posts with advanced filters, Timber get posts tax_query combining method is an essential tool in your WordPress toolbox.
In this article, we’ll dive deep into using Timber get_posts with tax_query, breaking down its syntax, usage, and providing real-world examples.
What is Timber get posts tax_query WordPress?
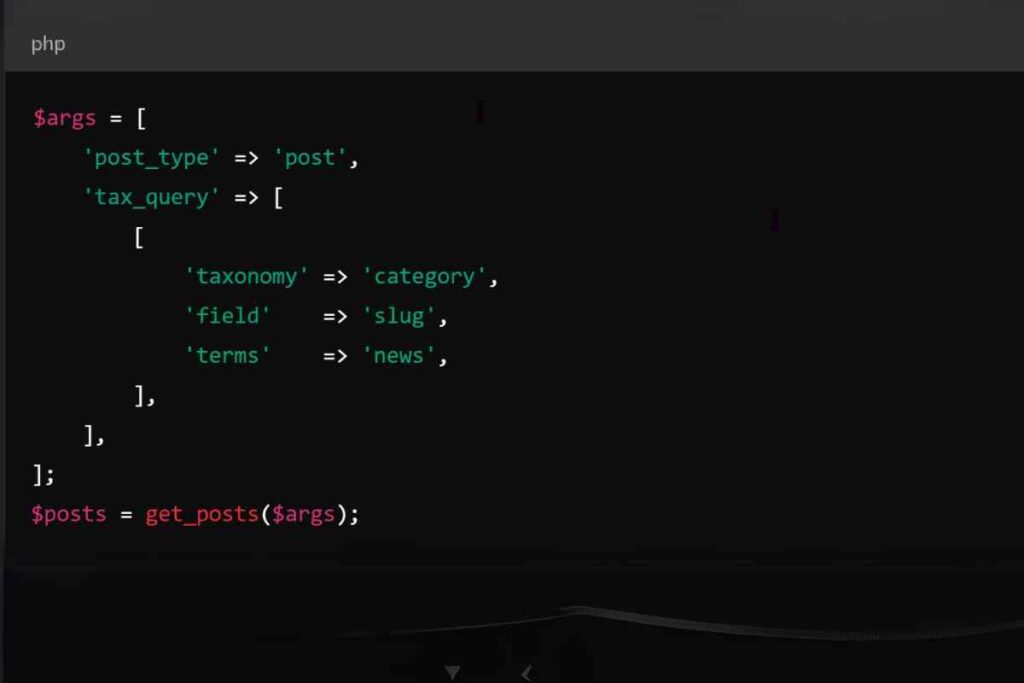
In WordPress, taxonomies are a way to group content. The most common taxonomies are categories and tags, but WordPress also supports custom taxonomies. tax_query is a powerful parameter that allows you to filter posts based on these taxonomies.
A Timber get posts tax_query can be used to query content by one or more taxonomy terms, making it a flexible way to fetch content. Here’s an example:
php
Copy code
$args = [
‘post_type’ => ‘post’,
‘tax_query’ => [
[
‘taxonomy’ => ‘category’,
‘field’ => ‘slug’,
‘terms’ => ‘news’,
],
],
];
$posts = get_posts($args);
The above code will fetch posts from the ‘news’ category. The tax_query enables filtering based on taxonomy relationships.
How Timber Simplifies WordPress Queries?
While WordPress’s built-in WP_Query is powerful, it can be verbose and somewhat complex.
Timber, however, simplifies querying posts and offers a cleaner, object-oriented approach.
When you use Timber get posts tax_query, you are working within its Timber::get_posts() method, which returns Timber\Post objects, making it easier to access post data directly in your Twig templates.
With Timber get_posts, you can pass the same query parameters you would with WP_Query, including tax_query, but with a more streamlined approach.
php
Copy code
use Timber\Timber;
$args = [
‘post_type’ => ‘post’,
‘tax_query’ => [
[
‘taxonomy’ => ‘category’,
‘field’ => ‘slug’,
‘terms’ => ‘events’,
],
],
];
$posts = Timber::get_posts($args);
In this case, Timber returns a collection of posts in the ‘events’ category. This is just the beginning of what you can do with Timber and tax_query.
Using Timber::get_posts with tax_query
The Timber::get_posts() method allows developers to retrieve WordPress posts based on specific criteria, including taxonomy terms.
By integrating tax_query, developers can filter content by categories, tags, or any custom taxonomies. Here’s an example of how to query posts using Timber and tax_query:
php
Copy code
use Timber\Timber;
$args = [
‘post_type’ => ‘post’,
‘tax_query’ => [
[
‘taxonomy’ => ‘category’,
‘field’ => ‘slug’,
‘terms’ => ‘technology’,
],
],
];
$posts = Timber::get_posts($args);
Timber::render(‘index.twig’, [‘posts’ => $posts]);
This code fetches posts belonging to the ‘technology’ category. Timber::get_posts simplifies the process of fetching content and passing it to a Twig template.
Syntax Breakdown of Timber get posts tax_query
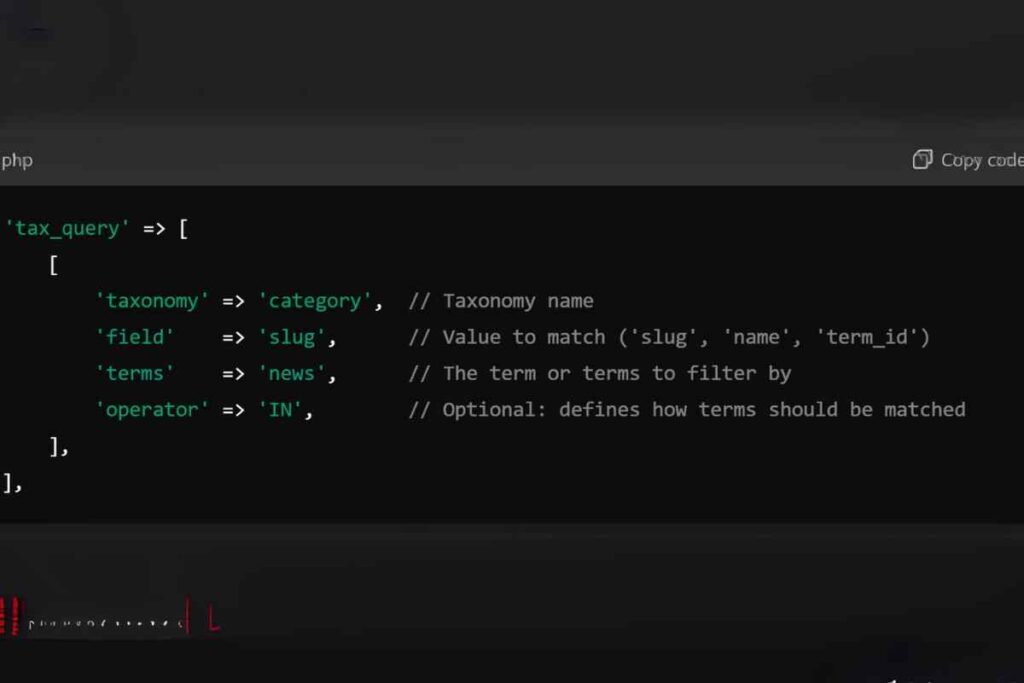
Understanding the syntax of Timber get posts tax_query is key to mastering its use. Here’s a breakdown of its structure:
php
Copy code
‘tax_query’ => [
[
‘taxonomy’ => ‘category’, // Taxonomy name
‘field’ => ‘slug’, // Value to match (‘slug’, ‘name’, ‘term_id’)
‘terms’ => ‘news’, // The term or terms to filter by
‘operator’ => ‘IN’, // Optional: defines how terms should be matched
],
],
Key Parameters
- taxonomy: The taxonomy you want to filter by, such as category, tag, or any custom taxonomy.
- field: The field used to match terms. Options include:
- term_id: Match by term ID.
- slug: Match by slug (the URL-friendly name).
- name: Match by term name.
- terms: The terms you want to query posts by.
- operator: Determines how the terms should be compared. Common options are:
- IN (default)
- NOT IN
- AND
Filtering by Multiple Taxonomies
One of the most powerful features of Timber get posts tax_query is the ability to filter by multiple taxonomies simultaneously. You can query posts that belong to both a specific category and tag, for example.
php
Copy code
$args = [
‘post_type’ => ‘post’,
‘tax_query’ => [
‘relation’ => ‘AND’, // Can also be ‘OR’
[
‘taxonomy’ => ‘category’,
‘field’ => ‘slug’,
‘terms’ => ‘technology’,
],
[
‘taxonomy’ => ‘post_tag’,
‘field’ => ‘slug’,
‘terms’ => ‘tutorial’,
],
],
];
$posts = Timber::get_posts($args);
In this example, Timber retrieves posts from both the ‘technology’ category and ‘tutorial’ tag. The relation parameter allows you to specify whether the filters should be combined with AND or OR.
Practical Examples of Timber get posts tax_query
Let’s go through a few practical examples to showcase how Timber get_posts with tax_query can be used in real-world scenarios.
Fetching Posts by Category
php
Copy code
$args = [
‘post_type’ => ‘post’,
‘tax_query’ => [
[
‘taxonomy’ => ‘category’,
‘field’ => ‘slug’,
‘terms’ => ‘news’,
],
],
];
$posts = Timber::get_posts($args);
This example retrieves posts from the ‘news’ category.
Using tax_query with Custom Taxonomies
php
Copy code
$args = [
‘post_type’ => ‘book’,
‘tax_query’ => [
[
‘taxonomy’ => ‘genre’,
‘field’ => ‘slug’,
‘terms’ => ‘fiction’,
],
],
];
$posts = Timber::get_posts($args);
This retrieves book posts in the ‘fiction’ genre.
Complex Queries with Multiple Taxonomies
php
Copy code
$args = [
‘post_type’ => ‘post’,
‘tax_query’ => [
‘relation’ => ‘OR’,
[
‘taxonomy’ => ‘category’,
‘field’ => ‘slug’,
‘terms’ => ‘travel’,
],
[
‘taxonomy’ => ‘post_tag’,
‘field’ => ‘slug’,
‘terms’ => ‘adventure’,
],
],
];
$posts = Timber::get_posts($args);
This query retrieves posts that belong to either the ‘travel’ category or ‘adventure’ tag.
Common Issues and Troubleshooting Tips
While working with Timber get_posts tax_query, developers may encounter a few common issues:
- Empty Results: Ensure that the taxonomy and term slugs are correct.
- Incorrect Field: If you’re using term_id, double-check that the term ID matches.
- Missing Terms: Verify that the terms you’re querying exist in your taxonomy.
Best Practices for SEO with Timber get posts tax_query

When using Timber get posts tax_query, you can boost your SEO by following best practices such as:
- Optimizing URLs: Ensure your term slugs are SEO-friendly.
- Using Descriptive Term Names: Use clear, keyword-rich names for categories and tags.
- Implementing Pagination: For large sets of results, use pagination to keep URLs clean and avoid content duplication.
Conclusion
In conclusion, Timber get posts tax_query is an essential tool for developers working with WordPress.
It simplifies the process of querying content based on taxonomy terms, allowing you to create powerful, flexible queries for your WordPress site.
By following the examples and best practices outlined in this guide, you can improve both your development process and your site’s SEO performance.
Whether you’re building a blog, e-commerce site, or custom WordPress theme, mastering Timber get_posts with tax_query is a crucial step toward achieving more dynamic and SEO-optimized results. Happy coding!
FAQs
What is Timber get posts tax_query in WordPress?
Timber get posts tax_query is used to filter posts based on taxonomy terms, such as categories or tags, allowing you to retrieve only relevant content.
How does Timber simplify tax_query usage?
Timber streamlines queries by using Timber::get_posts, making it easier to retrieve posts with taxonomy filters and pass them to Twig templates.
Can I filter by custom taxonomies in Timber?
Yes, Timber supports filtering posts by custom taxonomies using tax_query, just like default ones such as categories.
What are the main tax_query parameters?
The main parameters are taxonomy (taxonomy type), field (slug, term_id, name), and terms (terms to match).
How do I filter posts by multiple taxonomies?
Use the relation parameter in tax_query to filter by multiple taxonomies with an AND or OR condition.
What happens if I use an incorrect taxonomy in tax_query?
Using an incorrect taxonomy will result in no posts being returned. Ensure the taxonomy name is accurate.
Does tax_query affect performance on large sites?
tax_query can be performance-intensive on large sites. Use pagination and optimize your database to maintain speed.
Can I combine tax_query with other query parameters in Timber?
Yes, you can combine tax_query with other query parameters like post type or order for more complex queries.